使用原生js开发一个 轮播图、Tab 组件

轮播图和tab其实是一回事,都是实现内容区域的手动切换或自动轮换。
使用方式: 引入html模板,css,js文件, 实例化Tab构造函数即可,
例子以及参数:
new Tab({
triggerType: "click",// 触发切换的事件类型 click,mouseover 等事件
effect: '', //切换内容区域的动画 支持slide(滑动) fade("渐隐渐现")
show: 1,// 默认展示第几个
auto: 2000,//自动播放时间 单位毫秒 false为关闭自动播放
container: '.tab-wrapper1',// tab容器的class
});
在线Demo演示
jquery版本
demo: http://case.crazyming.com/?link=web/jq-tab
(由于jquery库对浏览器做了很多兼容处理,在低版本浏览器中推荐使用jquery版本)
原生js版本
demo: http://case.crazyming.com/?link=js-tab
实现步骤:
1.确定结构 编写html css
2.定义Tab构造函数 并将Tab挂载到window
3.判断 传入的参数 ,使用Object.assign 合并传入的参数
4.将用户传入的容器 作为 组件的根dom
5.在Tab原型添加show()方法,用于实现切换
6.在tab 的 按钮列表添加 事件监听,以实现tab的切换
7.在show方法中 根据 用户传入的effect 参数分别实现 切换动画
8.在Tab原型添加autoPlay()方法 使用定时器实现自动切换tab
9.根据用户传入的 auto 参数,来决定是否调用autoPlay()
10.根据用户传入的show参数 设置默认展示的索引
组件的HTML、CSS模板结构如下,就不多描述了:
<div class="tab-wrapper tab-wrapper1">
<ul class="tab-nav">
<li><a href="javascript:void(0)">互联网</a></li>
<li><a href="javascript:void(0)">数码</a></li>
<li><a href="javascript:void(0)">探索</a></li>
<li><a href="javascript:void(0)">科技</a></li>
</ul>
<div class="content-wrapper">
<div class="content-item "><img src="holder.js/500x200?text=互联网" alt=""></div>
<div class="content-item"><img src="holder.js/500x200?text=数码" alt=""></div>
<div class="content-item"><img src="holder.js/500x200?text=探索" alt=""></div>
<div class="content-item"><img src="holder.js/500x200?text=科技" alt=""></div>
</div>
</div>
.tab-wrapper {
width: 500px;
.tab-nav {
height: 30px;
display: flex;
background-color: #4095ff;
li {
flex: 1;
text-align: center;
list-style: none;
a {
display: block;
padding: 0 20px;
line-height: 30px;
text-decoration: none;
cursor: pointer;
color: white;
}
}
.active {
background-color: orangered;
}
}
.content-wrapper {
height: 200px;
background-color: white;
padding: 0;
overflow: hidden;
position: relative;
.content-item {
display: none;
width: 100%;
height: 100%;
overflow: hidden;
transition: all .3s;
position: absolute;
top: 0;
left: 0;
}
.current {
display: block;
}
}
}
;(function (window) {
function Tab(options) {
if (typeof options !== 'object') {
throw 'Tab参数错误';
}
//参数配置
this.options = Object.assign({
triggerType: "click",// 触发切换的事件类型 click,mouseover 等事件
effect: '', //切换动画:slide fade
show: 1,// 默认展示第几个
auto: false,//自动播放时间 单位秒 false 关闭自动播放
container: '.tab-wrapper',// tab容器的class
}, options);
//容器
this.dom = document.querySelector(this.options.container);
//tab 列表
this.tabUl = this.dom.querySelector('ul.tab-nav');
this.tabItems = this.dom.querySelectorAll('ul.tab-nav>li');
//tab 内容列表
this.contentItem = this.dom.querySelectorAll('div.content-wrapper>div.content-item');
// 用于自动轮播的计时器
this.timer = null;
var _this = this;
// 循环计数
_this.loop = 0;
//利用事件冒泡 在tab-nav 监听事件
this.tabUl.addEventListener(_this.options.triggerType, function (e) {
_this.show([].indexOf.call(_this.tabItems, e.target.parentNode));
});
if (!isNaN(_this.options.show)) {
_this.show(_this.options.show - 1);
}
if (_this.options.auto) {
_this.autoPlay();
_this.dom.addEventListener("mouseenter", function (e) {
clearInterval(_this.timer);
});
_this.dom.addEventListener("mouseleave", function (e) {
_this.autoPlay();
});
}
}
Tab.prototype = {
show: function (tabIndex) {
this.loop = tabIndex; //同步当前计数 用于自动播放
var _this = this;
[].forEach.call(this.tabItems, function (li, index) {
if (index === tabIndex) {
li.classList.add('active');
} else {
li.classList.remove("active");
}
});
[].forEach.call(this.contentItem, function (content, index) {
switch (_this.options.effect) {
case "fade":
content.classList.add('current');//让所有内容块都 display: block;
content.style.opacity = "0";
if (index === tabIndex) {
content.style.opacity = "1";
}
break;
case "slide":
content.classList.add('current');
if (index === tabIndex) {
var pre = _this.contentItem[index - 1] ? _this.contentItem[index - 1] : _this.contentItem[_this.contentItem.length - 1];//当上一项 content 不存在时 定义上一个 content 为 在最后一个content
var next = _this.contentItem[index + 1] ? _this.contentItem[index + 1] : _this.contentItem[0];//当下一项 content 不存在时 定义下一个 content 为 第一个content
pre.style.left = "-" + content.offsetWidth + "px";
next.style.left = content.offsetWidth + "px";
content.style.left = "0"
}
break;
default:
content.classList.remove('current');
if (index === tabIndex) {
content.classList.add('current');
}
}
})
},
autoPlay: function () {
if (!this.options.auto) return;
var tabLength = this.tabItems.length, _this = this;
_this.timer = setInterval(function () {
_this.loop++;
if (_this.loop >= tabLength) {
_this.loop = 0;
}
_this.show(_this.loop);
}, _this.options.auto);
}
};
window.Tab = Tab;
}
)(window);
版权声明:
作者:东明兄
链接:https://blog.crazyming.com/technology-sharing/1742/
来源:CrazyMing
文章版权归作者所有,未经允许请勿转载。
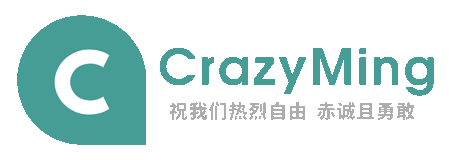
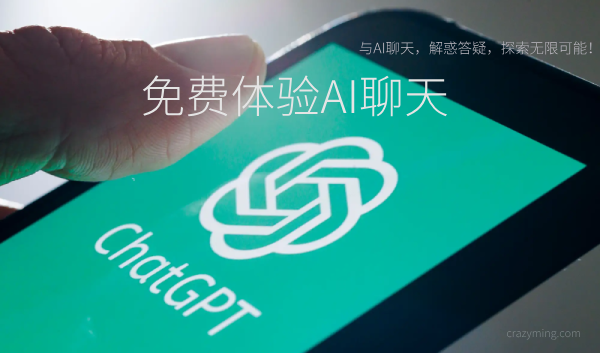
共有 0 条评论